آموزش ساخت برنامه چت با Firebase Cloud Messaging
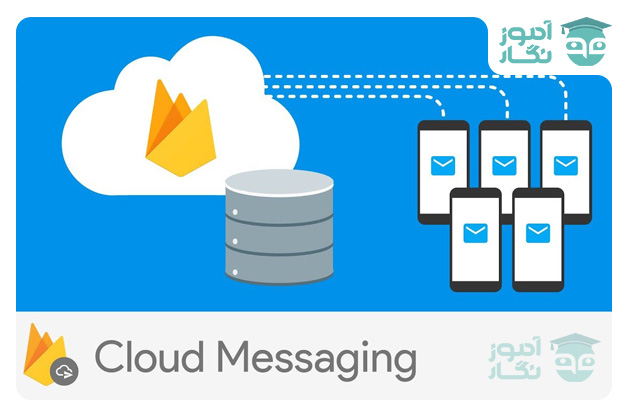
از جمله سرویس های خیلی کاربردی و رایگان که گوگل که برای ارسال Push Notification ایجاد کرده است سرویس FCM یا Firebase Cloud Messaging میباشد. این سرویس قبلا با نام GCM بود که به یکی از سرویس های Firebase اضافه شد.در این آموزش قصد داریم بطور کامل قدم به قدم طریقه سایت یک اپلیکیشن چت را با سرویس FCM پیاده سازی کنیم.
ایجاد پروژه جدید :
قبل از هرکاری میبایستی یک پروژه جدید در اندروید استودیو ایجاد کنید . در این مرحه گزینه New Project و سپس Empty project را انتخاب کنید.

در مرحله بعدی میبایستی اطلاعات اپلیکیشن مانند نام پروژه,نام PackageName و Minimum api را تعیین کنید.

سپس پروژه ایجاد می شود و می تواین شروع کنیم به ساخت پروژه چت.
اتصال برنامه به FireBase
از جمله ویژگی های خوب اندروید استودیو می توان به دسترسی به سرویس های گوگل اشاره کرد. یکی از سرویس های قابل دسترس Firebase میباشد. برای فعال کردن سرویس Firebase میبایستی از منوی Tools گزینه Firebase را انتخاب کرد

در مرحله میبایستی پروژه خود را با firebase setup کنید

سپس تنظیمات را مانند تصویر زیر اعمال نمایید.

در مرحله بعد میبایستی Dependency های لازمه را در فایل های gradle وارد نمایید.

به پروژهFirebase اضافه کردن سرویس
برای این کار یک کلاس میسازیم که سرویس فایربیس رو ارث بری میکنه :
import com.google.firebase.messaging.FirebaseMessagingService;
public class FirebaseService extends FirebaseMessagingService {
}
در مرحله بعد باید سرویس FirebaseService را در Manifest تعریف کنیم
برای اینکار قطعه کد زیر رو در تگ application اضافه می کنیم :
<service
android:name=".FirebaseService"
android:exported="false">
<intent-filter>
<action android:name="com.google.firebase.INSTANCE_ID_EVENT"/>
<action android:name="com.google.firebase.MESSAGING_EVENT"/>
</intent-filter>
</service>
در کلاس سرویس متد onMessageReceived رو override میکنیم:
هرزمان که پیام جدیدی دریافت کنیم پیام مورد نظر ما در قالب یک RemoteMessage در این متد به ما داده می شود.
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
public class FirebaseService extends FirebaseMessagingService {
@Override
public void onMessageReceived(@NonNull RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
}
}
برای شروع کار، مدل Message رو باید بسازیم :
و فیلدهای لازم رو براش مینویسیم.Message برای اینکار یک کلاس میسازیم به اسمه
ابتدا یک کلاس به نام Message می سازیم و فیلدهای لازم رو براش مینویسیم.
private String sender; رشته نام فرستنده پیام
private String content; رشته متن پیام
private boolean isMe;برای فهمیدن اینکه پیام دریافتی است یا ارسالی
private Date date; تاریخ پیام
import java.util.Date;
public class Message {
private String sender;
private String content;
private boolean isMe;
private Date date;
public Message(String sender, String content, boolean isMe, Date date) {
this.sender = sender;
this.content = content;
this.isMe = isMe;
this.date = date;
}
public String getSender() {
return sender;
}
public void setSender(String sender) {
this.sender = sender;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
public boolean getIsMe() {
return isMe;
}
public void setIsMe(boolean isMe) {
this.isMe = isMe;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
}
برای اینکه به مقادیر Context , Activity در همه قسمت های برنامه دسترسی داشته باشیم کلاس Application می سازیم.
import android.app.Activity;
import android.content.Context;
public class Application extends android.app.Application {
public static Context context;
public static Activity activity;
}
برای اینکه پیام دریافتی را از کلاس سرویس به کلاس های دیگر منتقل کنیم به interface نیاز داریم.
پس یک Interface با متد onReceivedMessage وبا ورودی Message برای دریافت پیام ایجاد می کنیم.
public interface onReceived {
void onReceivedMessage(Message message);
}
و در کلاس سرویس یک نمونه ازاین اینترفیس قرار می دهیم.
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
public class FirebaseService extends FirebaseMessagingService {
onReceived onReceived;
public void setOnReceived(onReceived onReceived) {
this.onReceived = onReceived;
}
@Override
public void onMessageReceived(@NonNull RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
}
}
حال وقت آن رسیده که اطلاعات مورد نیاز را از RemoteMessage دریافتی بگیریم و با آن یک نمونه Message بسازیم. و چک می کنیم اگر نام فرستنده پیام با نام کاربر یکی بود یعنی پیام دریافتی است پس بنابراین isMe را برابر True قرار می دهیم
و در نهایت از طریق اینترفیس پیام را به کلاسی که برای آن بدنه نوشته ارسال می کنیم.
بصورت زیر:
import android.content.SharedPreferences;
import androidx.annotation.NonNull;
import com.google.firebase.messaging.FirebaseMessagingService;
import com.google.firebase.messaging.RemoteMessage;
import java.util.Date;
import java.util.Map;
public class FirebaseService extends FirebaseMessagingService {
onReceived onReceived;
public void setOnReceived(com.example.chat.onReceived onReceived) {
this.onReceived = onReceived;
}
@Override
public void onMessageReceived(@NonNull RemoteMessage remoteMessage) {
super.onMessageReceived(remoteMessage);
Map<String, String> map = remoteMessage.getData();
long time = remoteMessage.getSentTime();
String sender = (String) map.values().toArray()[0];
String content = (String) map.values().toArray()[1];
SharedPreferences sh = Application.activity.getPreferences(MODE_PRIVATE);
String name = sh.getString("name", null);
boolean isme = name != null && name.equals(sender);
Message message = new Message(sender, content, isme, new Date(time));
if(onReceived != null){
onReceived.onReceivedMessage(message);
}
}
}
طراحی رابط کاربری activity_main.xml
یک EditText برای دریافت نام کاربر و یکی برای تاپیک مورد نظر برای چت و یک دکمه برای عضو شدن در
تاپیک قرار می دهیم.
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/back_blue"
tools:context=".MainActivity">
<EditText
android:id="@+id/edt_name"
android:layout_width="match_parent"
android:layout_height="60dp"
android:layout_marginStart="12dp"
android:layout_marginTop="200dp"
android:layout_marginEnd="12dp"
android:hint="Name"
android:textColorHint="@color/light_gray"
android:textColor="@color/light_gray"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.666"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/edt_topic"
android:layout_width="match_parent"
android:layout_height="60dp"
android:layout_margin="12dp"
android:hint="Topic"
android:textColorHint="@color/light_gray"
android:textColor="@color/light_gray"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.666"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/edt_name" />
<Button
android:id="@+id/btn_subscribe"
android:layout_width="match_parent"
android:layout_height="60dp"
android:layout_margin="12dp"
android:text="Subscribe"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.666"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/edt_topic" />
</androidx.constraintlayout.widget.ConstraintLayout>
یک Activity به نام ChatActivity اضافه می کنیم برای قسمت چت برنامه که این اکتیویتی به نام کاربر و تاپیک Subscribe شده نیاز دارد.
بنابراین نام کاربر را در SharedPreferences ذخیره می کنیم و این دو فیلد را به اکتیویتی بعد انتقال می دهیم.
نام کاربر را برای متوجه شدن ارسالی یا دریافتی بودن پیام در SharedPreferences ذخیره می کنیم.
و برای ارسال پیام به تاپیک نام کاربر و نام تاپیک را نیاز داریم که آن را انتقال می دهیم.
MainActivity کد
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
import com.google.firebase.messaging.FirebaseMessaging;
public class MainActivity extends AppCompatActivity {
EditText edtName, edtTopic;
Button btnSubscribe;
SharedPreferences sh;
SharedPreferences.Editor editor;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
edtName = findViewById(R.id.edt_name);
edtTopic = findViewById(R.id.edt_topic);
btnSubscribe = findViewById(R.id.btn_subscribe);
sh = getPreferences(MODE_PRIVATE);
editor = sh.edit();
String name = sh.getString("name", "");
if (!name.equals("")) {
edtName.setText(name);
btnSubscribe.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (edtTopic.getText().toString().isEmpty() && !edtName.getText().toString().isEmpty()) {
Bundle bundle = new Bundle();
editor.putString("name", edtName.getText().toString());
editor.apply();
try {FirebaseMessaging.getInstance().subscribeToTopic(edtTopic.getText().toString());
Intent intent = new Intent(getApplication(), ChatActivity.class);
intent.putExtra("topic", edtTopic.getText().toString());
intent.putExtra("name", edtName.getText().toString());
startActivity(intent);
} catch (Exception e) {
Toast.makeText(getApplicationContext(), "Can not subscribe", Toast.LENGTH_SHORT).show();
}} else {
Toast.makeText(getApplicationContext(), "Please fill all fields", Toast.LENGTH_SHORT).show();
}
}
});
}
}
}
در MainActivity، Subscribe می کنیم و اگر موفقیت آمیز بود به اکتیویتی چت می رویم.
حالا نوبت اضافه کردن طراحی پیام است.
بنابراین در پوشه drawable دو Shape برای پیام های دریافتی و ارسالی ایجاد می کنیم.
income_bubble_shapeپیام های دریافتی
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<rotate
android:fromDegrees="-45"
android:pivotX="0%"
android:pivotY="0%"
android:toDegrees="0" >
<shape android:shape="rectangle" >
<solid android:color="@color/income_blue" />
</shape>
</rotate>
</item>
<item android:left="16dp">
<shape android:shape="rectangle" >
<solid android:color="@color/income_blue" />
<corners android:radius="14dp" />
</shape>
</item>
</layer-list>
outgoing_bubble_shapeپیام های ارسالی
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item>
<rotate
android:fromDegrees="45"
android:pivotX="100%"
android:pivotY="0%"
android:toDegrees="0">
<shape android:shape="rectangle">
<solid android:color="@color/out_blue" />
</shape>
</rotate>
</item>
<item android:right="16dp">
<shape android:shape="rectangle">
<solid android:color="@color/out_blue" />
<corners android:radius="14dp" />
</shape>
</item>
</layer-list>
برای نشان دادن پیام ها در RecyclerView به یک Adapter نیاز داریم.
و برای Adapter به دو فایل Xml برای پیام های ارسالی و دریافتی نیاز داریم.
layout پیام های دریافتی Received_message
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginEnd="8dp"
android:background="@color/back_blue"
android:padding="8dp">
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/main_layout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@drawable/income_bubble_shape"
android:paddingLeft="24dp"
android:paddingTop="2dp"
android:paddingRight="5dp"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/txt_sender"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@color/out_blue"
android:textSize="16sp"
android:textStyle="bold"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@id/txt_sender">
<TextView
android:id="@+id/txt_content"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="6dp"
android:maxWidth="230dp"
android:minWidth="70dp"
android:paddingLeft="6dp"
android:paddingTop="4dp"
android:paddingRight="6dp"
android:textColor="@color/light_gray"
android:textSize="16sp" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
<TextView
android:id="@+id/txt_time"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="4dp"
android:textColor="@color/light_gray"
android:textSize="10sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toEndOf="@id/main_layout" />
</androidx.constraintlayout.widget.ConstraintLayout>
layout پیام های ارسالی Sent_message
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="@color/back_blue"
android:padding="2dp"
android:layout_marginEnd="8dp"
android:layout_marginTop="8dp">
<androidx.constraintlayout.widget.ConstraintLayout
android:id="@+id/main_layout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingRight="15dp"
android:background="@drawable/outgoing_bubble_shape"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="vertical"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintTop_toTopOf="parent">
<TextView
android:id="@+id/txt_content_me"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="8dp"
android:maxWidth="230dp"
android:paddingLeft="12dp"
android:paddingTop="8dp"
android:paddingRight="12dp"
android:minWidth="50dp"
android:textColor="@color/light_gray"
android:textSize="16sp" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
<TextView
android:id="@+id/txt_time_me"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@color/light_gray"
android:textSize="10sp"
android:layout_marginEnd="4dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/main_layout" />
</androidx.constraintlayout.widget.ConstraintLayout>
Activity_chat.xml طراحی رابط کاربری
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/back_blue"
tools:context=".ChatActivity">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recycler"
android:layout_width="match_parent"
android:layout_height="0dp"
android:background="@color/back_blue"
app:layout_constraintBottom_toTopOf="@+id/edt_content"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<EditText
android:id="@+id/edt_content"
android:layout_width="0dp"
android:layout_height="55dp"
android:hint="Message"
android:layout_marginStart="12dp"
android:textColor="@color/light_gray"
android:textColorHint="@color/light_gray"
android:background="@color/back_blue"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/btn_send"
app:layout_constraintStart_toStartOf="parent" />
<ImageButton
android:id="@+id/btn_send"
android:layout_width="55dp"
android:layout_height="55dp"
android:src="@drawable/ic_baseline_send_24"
android:background="@color/back_blue"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
در کلاسAdapter به متد getItemViewType نیاز داریم تا متوجه شویم پیام مورد نظر ارسالی بوده یا دریافتی.
کلاس آداپتر MessageAdapter.java
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.recyclerview.widget.RecyclerView;
import java.util.ArrayList;
public class MessageAdapter extends RecyclerView.Adapter {
private ArrayList<Message> messages;
private static final int VIEW_TYPE_MESSAGE_SENT = 0;
private static final int VIEW_TYPE_MESSAGE_RECEIVE = 1;
@Override
public int getItemViewType(int position) {
Message message = messages.get(position);
if (message.getIsMe())
return VIEW_TYPE_MESSAGE_SENT;
return VIEW_TYPE_MESSAGE_RECEIVE;
}
public MessageAdapter(ArrayList<Message> messages) {
this.messages = messages;
}
public void addMessage(Message messages) {
this.messages.add(messages);
notifyDataSetChanged();
}
@NonNull
@Override
public RecyclerView.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view;
if (viewType == VIEW_TYPE_MESSAGE_SENT) {
view = LayoutInflater.from(parent.getContext()).inflate(R.layout.sent_message, parent, false);
return new SentVH(view);
} else if (viewType == VIEW_TYPE_MESSAGE_RECEIVE) {
view = LayoutInflater.from(parent.getContext()).inflate(R.layout.received_message, parent, false);
return new ReceiveVH(view);
}
return null;
}
@Override
public void onBindViewHolder(@NonNull RecyclerView.ViewHolder holder, int position) {
Message message = messages.get(position);
switch (holder.getItemViewType()) {
case VIEW_TYPE_MESSAGE_SENT:
((SentVH) holder).bind(message);
break;
case VIEW_TYPE_MESSAGE_RECEIVE:
((ReceiveVH) holder).bind(message);
}
}
@Override
public int getItemCount() {
return messages.size();
}
}
class SentVH extends RecyclerView.ViewHolder {
TextView txtContent, txtTime;
public SentVH(View itemView) {
super(itemView);
txtContent = itemView.findViewById(R.id.txt_content_me);
txtTime = itemView.findViewById(R.id.txt_time_me);
}
public void bind(Message message) {
txtContent.setText(message.getContent());
txtTime.setText(message.getDate().getHours() + ":" + message.getDate().getMinutes());
}
}
class ReceiveVH extends RecyclerView.ViewHolder {
TextView txtSender, txtContent, txtTime;
public ReceiveVH(View itemView) {
super(itemView);
txtSender = itemView.findViewById(R.id.txt_sender);
txtContent = itemView.findViewById(R.id.txt_content);
txtTime = itemView.findViewById(R.id.txt_time);
}
public void bind(Message message) {
txtSender.setText(message.getSender());
txtContent.setText(message.getContent());
txtTime.setText(message.getDate().getHours() + ":" + message.getDate().getMinutes());
}
}
به سایت Firebase مراجعه کرده و طبق مراحل زیر Api Key را بدست می آوریم.برای اینکار از کنسول فایربیس در بخش project overview گزینه Project Settings را انتخاب کنید.

در صفحه بعدی وارد تب Cloud Messaging شده و مقدار Server Key را کپی کنید.

و برای ارسال پیام یک کلاس می نویسیم :
SendMessage.java
private class sendMessage extends AsyncTask<String, Void, Integer> {
@Override
protected Integer doInBackground(String... strings) {
final String KEY = "YOUR_API_KEY"; // change this to your api key
String endpoint = "https://fcm.googleapis.com/fcm/send";
String title = strings[0];
String content = strings[1];
String topic = strings[2];
try {
URL url = new URL(endpoint);
HttpsURLConnection httpsURLConnection = (HttpsURLConnection) url.openConnection();
httpsURLConnection.setReadTimeout(10000);
httpsURLConnection.setConnectTimeout(15000);
httpsURLConnection.setRequestMethod("POST");
httpsURLConnection.setDoInput(true);
httpsURLConnection.setDoOutput(true);
httpsURLConnection.setRequestProperty("authorization", "key=" + KEY);
httpsURLConnection.setRequestProperty("Content-Type", "application/json");
JSONObject body = new JSONObject();
JSONObject data = new JSONObject();
data.put("title", title);
data.put("content", content);
body.put("data", data);
body.put("to", "/topics/" + topic);
OutputStream outputStream = new BufferedOutputStream(httpsURLConnection.getOutputStream());
BufferedWriter writer = new BufferedWriter(new OutputStreamWriter(outputStream, "utf-8"));
writer.write(body.toString());
writer.flush();
writer.close();
outputStream.close();
int responseCode = httpsURLConnection.getResponseCode();
String responseMessage = httpsURLConnection.getResponseMessage();
Log.d("asdasd", responseCode + " : " + responseMessage);
InputStream inputStream = null;
if (responseCode >= 400 && responseCode <= 499) {
inputStream = httpsURLConnection.getErrorStream();
issues.onError();
} else {
inputStream = httpsURLConnection.getInputStream();
}
String result = inputStream.toString();
Log.e("asdasd", result);
if (responseCode == 200) {
Log.e("asdasd", "Success sent message");
issues.onSuccess();
} else {
Log.e("asdasd", "Error Response");
issues.onError();
}
} catch (Exception ex) {
Log.e("asdasd", ex.toString());
issues.onError();
}
return null;
}
}
ChatActivity و در نهایت کد
public class ChatActivity extends AppCompatActivity {
String name, topic;
EditText edtContent;
ImageButton btnSend;
MessageAdapter adapter;
RecyclerView recyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_chat);
edtContent = findViewById(R.id.edt_content);
btnSend = findViewById(R.id.btn_send);
recyclerView = findViewById(R.id.recycler);
Bundle bundle = getIntent().getExtras();
name = bundle.getString("name");
topic = bundle.getString("topic");
adapter = new MessageAdapter(new ArrayList<>());
recyclerView.setLayoutManager(new LinearLayoutManager(getApplicationContext(), RecyclerView.VERTICAL, false));
recyclerView.setAdapter(adapter);
btnSend.setOnClickListener(v -> {
sendMessage message = new sendMessage();
message.execute(name, edtContent.getText().toString(), topic);
edtContent.setText("");
});
FirebaseService.setOnReceived(new onReceived() {
@Override
public void onReceivedMessage(Message message) {
runOnUiThread(new Runnable() {
@Override
public void run() {
adapter.addMessage(message);
}
});
}
});
}
}
در نهایت خروجی زیر را مشاهده می کنید.

نتیجه گیری
همچنان که مشاهده کردید فایربیس یکی از سرویس های بسیار مفید برای برنامه نویسان اندروید است که می توانید با استفاده از این سرویس ها در اپلیکیشن های موبایل خود , از امکانات رایگان فایربیس استفاده کنید. خیلی خوشحال میشم نظر خودتون رو در مورد این آموزش با ما در میان بگذارید.
دیدگاهتان را بنویسید