استفاده از Retrofit در ViewModel اندروید
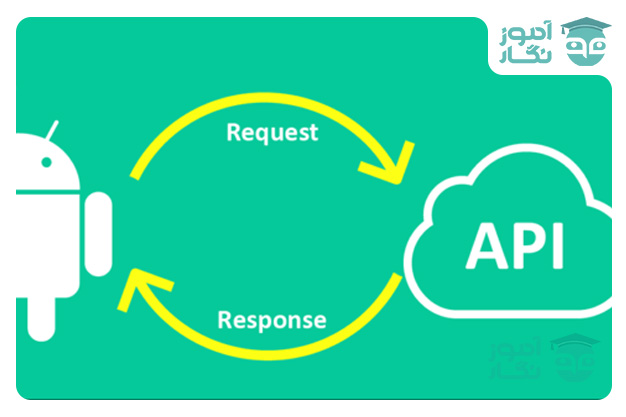
یک آموزش ساده
درباره ViewModel در اندروید شنیده اید؟ ViewModel اندروید جزء معماری است که برای ذخیره داده های مربوط به رابط کاربری طراحی شده است.باتوجه به وب سایت Android Developer.
کلاس ViewModel برای ذخیره و مدیریت داده های مربوط به رابط کاربری به روش آگاهانه در یک چرخه حیات طراحی شده است. کلاس ViewModel
اجازه می دهد تا داده ها بعد از تغییرات پیکربندی مانند چرخش صفحه باقی بماند.
اگر شما یکی از آخرین آموزش Retrofit ما درباره دریافت داده های JSON از یک URL به یاد آورید . اکنون اگر یکی از فعالیت های ما داده های مربوط به آن را از سرور دریافت کند ، اما اگر به دلایلی (به عنوان مثال تغییر جهت) فعالیت دوباره ایجاد می شود و داده ها را دوباره از سرور دریافت می کند . دریافت داده مشابه از سرور باعث اتلاف منابع می شود . یک راه حل کارآمد در اینجا جدا کردن مالکیت داده نمایش از منطق کنترل کننده UI است.
بنابراین ، در این آموزش Android View Model همان کاری را که در آموزش Retrofit قبلی انجام دادیم انجام خواهیم داد اما در اینجا از معماری ViewModel استفاده خواهیم کرد.
راه اندازی پروژه اندروید با RecyclerView و Retrofit
ایجاد یک پروژه جدید اندروید استودیو
- مثل همیشه اولین قدم ایجاد یک پروژه جدید Android Studio است. و در آن پروژه نحوه کار با Android ViewModel را خواهیم دید و در آن پروژه نحوه کار با Android ViewModel را خواهیم دید.
- من یک پروژه با استفاده از EmptyActivity به نام AndroidViewModel ایجاد کرده ام.
API برای دریافت داده ها
- در صورت داشتن هر URL می توانید برای دریافت داده ها استفاده کنید. در اینجا قصد دارم از URL زیر استفاده کنم.
- URL بالا لیستی از Marvel Super Heroes را برمی گرداند. می توانید URL را باز کنید تا ببینید چه چیزی در حال بازگشت است. این همان نشانی اینترنتی است که هنگام یادگیری Retrofit JSON از آن استفاده کردیم.
افزودن وابستگی مورد نیاز
برای این پروژه ما می خواهیم از وابستگی های زیر استفاده کنیم.
- RecyclerView – superheroes برای نمایش لیستی از
- Glide – URL برای بارگیری تصویر از.
- Retrofit – URL برای دریافت داده از.
- ViewModel – جزء معماری است.
بنابراین ابتدا تمام وابستگی های ذکر شده در بالا را در فایل build.gradle سطح App اضافه خواهیم کرد.
dependencies {
implementation fileTree(include: ['*.jar'], dir: 'libs')
implementation 'com.android.support:appcompat-v7:27.1.1'
implementation 'com.android.support.constraint:constraint-layout:1.1.0'
testImplementation 'junit:junit:4.12'
androidTestImplementation 'com.android.support.test:runner:1.0.2'
androidTestImplementation 'com.android.support.test.espresso:espresso-core:3.0.2'
//adding dependencies
implementation 'com.android.support:recyclerview-v7:27.1.1'
implementation 'com.squareup.retrofit2:retrofit:2.4.0'
implementation 'com.squareup.retrofit2:converter-gson:2.4.0'
implementation 'com.github.bumptech.glide:glide:4.3.1'
annotationProcessor 'com.github.bumptech.glide:compiler:4.3.1'
implementation "android.arch.lifecycle:extensions:1.1.0"
implementation "android.arch.lifecycle:viewmodel:1.1.0"
}
پس از اضافه کردن همه وابستگی ها پروژه تان را sync کنید .
ایجاد کلاس POJO برای Hero
- یک کلاس جدید به نام java ایجاد کنید و کد زیر را بنویسید.
package com.example.belalkhan.androidviewmodel;
public class Hero {
private String name;
private String realname;
private String team;
private String firstappearance;
private String createdby;
private String publisher;
private String imageurl;
private String bio;
public Hero(String name, String realname, String team, String firstappearance, String createdby, String publisher, String imageurl, String bio) {
this.name = name;
this.realname = realname;
this.team = team;
this.firstappearance = firstappearance;
this.createdby = createdby;
this.publisher = publisher;
this.imageurl = imageurl;
this.bio = bio;
}
public String getName() {
return name;
}
public String getRealname() {
return realname;
}
public String getTeam() {
return team;
}
public String getFirstappearance() {
return firstappearance;
}
public String getCreatedby() {
return createdby;
}
public String getPublisher() {
return publisher;
}
public String getImageurl() {
return imageurl;
}
public String getBio() {
return bio;
}
}
راه اندازی RecyclerView
ایجاد RecyclerView و RecyclerView Layout
- حالا وارد xml شوید و یک RecyclerView در اینجا ایجاد کنید ، در این RecyclerView ما لیست Hero را نمایش خواهیم داد.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/recyclerview"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
- اکنون همچنین یک فایل منبع layout دیگر برای RecyclerView ایجاد خواهیم کرد .
- یک فایل منبع layout به نام xml ایجاد کنید و کد xml زیر را بنویسید.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView"
android:layout_width="match_parent"
android:layout_height="200dp" />
<TextView
android:id="@+id/textView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textAlignment="center"
android:textAppearance="@style/Base.TextAppearance.AppCompat.Large" />
</LinearLayout>
ایجاد آداپتور RecyclerView
- اکنون ما به آداپتور RecyclerView نیاز داریم. ما قصد داریم از کد زیر برای این کار استفاده کنیم. اگر مطمئن نیستید که در مورد چه چیزی صحبت می کنید ، می توانید آموزش RecyclerView را بررسی کنید.
- بنابراین کافی است یک کلاس جدید به نام java ایجاد کنید و کد زیر را بنویسید.
package com.example.belalkhan.androidviewmodel;
import android.content.Context;
import android.support.annotation.NonNull;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.bumptech.glide.Glide;
import java.util.List;
public class HeroesAdapter extends RecyclerView.Adapter<HeroesAdapter.HeroViewHolder> {
Context mCtx;
List<Hero> heroList;
public HeroesAdapter(Context mCtx, List<Hero> heroList) {
this.mCtx = mCtx;
this.heroList = heroList;
}
@NonNull
@Override
public HeroViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(mCtx).inflate(R.layout.recyclerview_layout, parent, false);
return new HeroViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull HeroViewHolder holder, int position) {
Hero hero = heroList.get(position);
Glide.with(mCtx)
.load(hero.getImageurl())
.into(holder.imageView);
holder.textView.setText(hero.getName());
}
@Override
public int getItemCount() {
return heroList.size();
}
class HeroViewHolder extends RecyclerView.ViewHolder {
ImageView imageView;
TextView textView;
public HeroViewHolder(View itemView) {
super(itemView);
imageView = itemView.findViewById(R.id.imageView);
textView = itemView.findViewById(R.id.textView);
}
}
}
راه اندازی RecyclerView
- حالا به java برگردید و کدهای زیر را بنویسید.
public class MainActivity extends AppCompatActivity {
RecyclerView recyclerView;
HeroesAdapter adapter;
List<Hero> heroList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
recyclerView = findViewById(R.id.recyclerview);
recyclerView.setHasFixedSize(true);
recyclerView.setLayoutManager(new LinearLayoutManager(this));
}
}
- اکنون ما Retrofit را برای دریافت داده ها از URL تنظیم می کنیم.
راه اندازی Retrofit
The API Interface
- یک interface جید java ایجاد کنید .
package com.example.belalkhan.androidviewmodel;
import java.util.List;
import retrofit2.Call;
import retrofit2.http.GET;
public interface Api {
String BASE_URL = "https://simplifiedcoding.net/demos/";
@GET("marvel")
Call<List<Hero>> getHeroes();
}
- تا اینجا ما کارهایی را که قبلاً در آموزشهای قبلی آموخته ایم ، انجام می دهیم. اکنون برای بارگذاری داده ها در RecyclerView از معماری ViewModel استفاده خواهیم کرد.
استفاده از Android ViewModel برای بارگذاری همزمان داده ها با استفاده از Retrofit
ایجاد ViewModel اندروید
- برای ایجاد ViewModel ، باید یک کلاس ایجاد کنیم که از کلاس ViewModel ارث بری کند.
- در اینجا من یک کلاس به نام java ایجاد می کنم.
package com.example.belalkhan.androidviewmodel;
import android.arch.lifecycle.LiveData;
import android.arch.lifecycle.MutableLiveData;
import android.arch.lifecycle.ViewModel;
import android.widget.Toast;
import java.util.List;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
public class HeroesViewModel extends ViewModel {
//this is the data that we will fetch asynchronously
private MutableLiveData<List<Hero>> heroList;
//we will call this method to get the data
public LiveData<List<Hero>> getHeroes() {
//if the list is null
if (heroList == null) {
heroList = new MutableLiveData<List<Hero>>();
//we will load it asynchronously from server in this method
loadHeroes();
}
//finally we will return the list
return heroList;
}
//This method is using Retrofit to get the JSON data from URL
private void loadHeroes() {
Retrofit retrofit = new Retrofit.Builder()
.baseUrl(Api.BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build();
Api api = retrofit.create(Api.class);
Call<List<Hero>> call = api.getHeroes();
call.enqueue(new Callback<List<Hero>>() {
@Override
public void onResponse(Call<List<Hero>> call, Response<List<Hero>> response) {
//finally we are setting the list to our MutableLiveData
heroList.setValue(response.body());
}
@Override
public void onFailure(Call<List<Hero>> call, Throwable t) {
}
});
}
}
- دریافت (واکشی) با استفاده از قسمت Retrofit در اینجا دقیقاً همان چیزی است که قبلاً در آموزش Retrofit آموخته ایم.
- و نکته ViewModel بسیار آسان است ، ما فقط متد به نام getHeroes () داریم. در داخل این متد داریم بررسی می کنیم که آیا لیست null است ، بنابراین ما آن را به صورت غیرهم زمان دریافت خواهیم کرد. بخاطر بسپارید که برای ذخیره سازی لیست باید از کلاسهای MutableLiveData و LiveData استفاده کنیم و مابقی را با جزیی از ViewModel مدیریت می کند. بنابراین نیازی نیست نگران موارد دیگر باشید.
استفاده از ViewModel اندروید برای نمایش لیست Hero
- کد زیر را در انتهای متد onCreate () در java بنویسید.
HeroesViewModel model = ViewModelProviders.of(this).get(HeroesViewModel.class);
model.getHeroes().observe(this, new Observer<List<Hero>>() {
@Override
public void onChanged(@Nullable List<Hero> heroList) {
adapter = new HeroesAdapter(MainActivity.this, heroList);
recyclerView.setAdapter(adapter);
}
});
اکنون می توانید برنامه خود را اجرا کنید ، بررسی کنید که آیا کار می کند یا خیر.

همانطور که می بینید خوب کار می کند. مزیت انجام این روش این است که اگر فعالیت ما دوباره ایجاد شود ، داده ها را دوباره به دست نمی آورد و باعث کارآیی بیشتر برنامه ما می شود. بله ، شما می توانید کد منبع من را از مخزن GitHub زیر دریافت کنید.
https://simplified-coding-downloads.firebaseapp.com/download.html?id=-M-k65o_-mgZyViuAPRE
دیدگاهتان را بنویسید